Hello dear visitor !!! You all the again most welcome to this domain bittutech.com . Today we are going to discus about most important concept of Python Programming Language which is File Handling including What is file?, Types of files, Modes of files, Opening and Closing of file, Working with files… and many more. So let’s dive in this amazing concept ๐.
Most of the programming languages works with files because files helps in storing information permanently.
What is File?
Table of Contents
A file in itself is a bunch of bites stored on some storage devices like hard disk, thumb drive or pen drive.
Data file
The data files are the files that stored data pertaining to a specific application for later use. The data files can be stored in two ways.
1. Text Files
A text files stores information in the form of a streamed of ASCII or UNICODE character coding system. In Python Programming Language each line of text is terminated, delimited with a special character known as EOL (End Of Line). In python the ‘EOL’ character is the new line character (\n).
The text file can be following types :
- Regular text file:- These are text file which store the text in same form as typed. Extension of this types of file is .txt .
- Delimited text file:- these text files uses specific character that are used to separate the values. Tab and Comma are used to separate the value.
NOTE 1:- If our files are separated through the Tab character, so the files are known as Tab Separated Files(.txt or .csv) .
NOTE 2:- The files that are separated through the Comma character, so the file is known as Comma Separated File (.csv) .
NOTE 3:- The CSV(Comma Separated Values) format is a popular import and export for spreadsheet and database. Most commonly used delimiter in a CSV File is Comma(,), but it can also use other delimiter character like Tab(–>), Tilde(~).
NOTE 4:- Some setup files (e.g., INI files and Rich Text Format files(.RTF)) are also text files.
2. Binary Files
A binary file stores the informations in the form of stream of Bytes.
A binary files contains information in the same format in which the information is held in the memory. This file content that is return to you is row(with no translation or no specific encoding). Binary Files are the best way to store program informations.
NOTE:- The Text Files can be opened in any text editor and are in human readable form while Binary Files are not in human readable form.
Opening and Closing Files
We need to open it in a specific mode as per the file manipulation task you want to perform. The most basic file manipulation task including adding, modifying or deleting data in a file.
- Reading data from files.
- Writing data to files.
- Appending data to files.
OPENING FILES
In data file handling through Python the first thing that we need to open the file. It is done by using open() function.
SYNTAX;-
<file_ object name> = open(file name)
For Example:-
f = open("data.txt","r")
NOTE:- A file object is known as file handle.
NOTE:- Python’s open() function creates a file object which serves as a link to file residing on your computer. The first parameter defines location or path of the file and second parameter defines the mode of opening the file (read or write mode).
The default file open mode is read mode i.e., if you do not provide any file mode ,Python will open it in read mode (“r”).
File Object/File Handle: File Object are need to read and write data to a file on disk. The File Object is used to obtain a reference to the file on disk and open it for a number of different tasks.
A File Object of Python is stream of bytes where the data cab be read either byte by byte or line by line or collectively.
File Access Modes
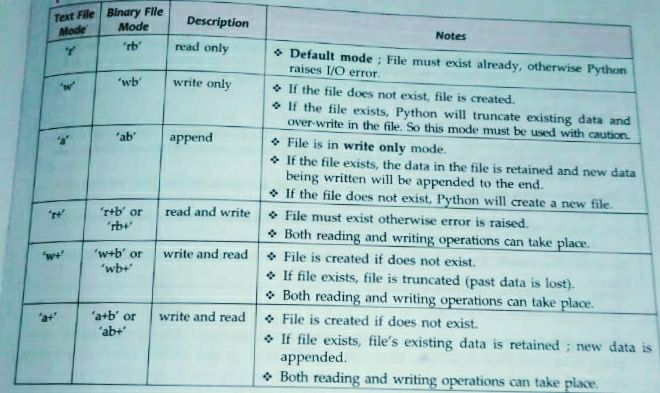
A file-mode governs the type of operations (e.g., read/write/append ) possible in the opened file i.e., it refers to how the file will be used once it’s opened.
NOTE:- Adding ‘b’ to the text- file mode makes it binary- file mode.
CLOSING FILES
An open file is closed by the calling the close() method of its file- object.
A close() function breaks the link of file- object and the file on the disk. After close(), no tasks can be performed on the file through the file-object (or file- handle).
SYNTAX;
<fileHandle>.close()
For Example;
If a file data.txt is opened via file-handle f , it will closed by…๐
f.close()
Working with Text Files
1. Reading from Text Files
Python provides mainly three types of read functions to read from a data file.
I) read( ) :- This function read at most ‘n’ bytes; if no ‘n’ is specified, reads the entire file. This function returns the read bytes in the form of a string.
SYNTAX:-
<filehandle>.read( [n] )
For Example:-
Reading a file’s first 30 bytes and painting it.
myfile = open("poem.txt","r")
str = myfile.read(30)
print(str)
II) readline( ) :- This function reads a line. If ‘n’ is specified reads at most ‘n’ bytes. This function returns the read bytes in the form of a string ending with line character or returns a blank string if no more bytes are left for reading in the file.
SYNTAX:-
<file-handle>.readline([n])
For Example:-
Reading a file’s first three lines – line by line.
with open('file.txt', 'r') as f:
for i in range(3):
line = f.readline()
print(line.strip())
Explanation:
1. `with open(‘file.txt’, ‘r’) as f`: Opens the file in read mode and assigns it to the variable `f`.
2. `for i in range(3)`: Loops three times to read the first three lines.
3. `line = f.readline()`: Reads a line from the file.
4. `print(line.strip())`: Prints the line after removing any trailing newlines or whitespace using the `strip()` method.
Note: Make sure to replace `’file.txt’` with the actual file path and name you want to read.
III) readlines( ) :- This function reads all lines and returns them in a list.
SYNTAX:-
<file-handle>.readlines()
For Example:-
Reading the complete file in a list.
f = open('file.txt', 'r')
file_list = f.readlines( )
print(file_list)
f.close( )
2. Writing onto Text Files
After working with file reading functions, let us talk about the writing functions for data files available in Python. Like reading functions, the writing functions also work on open files, i.e., the files that are opened and linked via a file-object or file-handle.
Python provides two types of write functions –
I) write( ) :-This function writes string ‘str1’ to file referenced by <file-handle>
SYNTAX:-
<file-handle>.write(str1)
II) writelines( ) :- This function writes all strings in list ‘L’ as lines to file referenced by <file-handle>.
SYNTAX:-
<file-handle>.writelines(L)
3. Appending a File
A file opened in “a” or append mode retains its previous data while allowing you to add newer data into.
When you open a file in “w” or write mode python overwrites an existing file or creates a non-existing file. That means, for an existing file with the same name, the earlier data get lost. If, however, you want to write into the file without retaining the old data, then you should open the file in “a” or append mode.
You can also add a plus symbol(+) with file read mode to facilitate reading as well as writing.
For Example:-
Create a file to hold done data.
fout=open("Student.dat","w")
for i in range(5):
Name=input("enter name of students:-")
fout.write(Name)
fout.close()
The flush( ) function
When you write onto a file using any of the write functions, Python holds everything to write in the file in buffer and pushes it on to actual file on storage device a later time. If however, you want to force Python to write the contents of uffer onto storage, you can use flush( ) function.
Python automatically flushes the file buffers when closing them i.e., this function is implicity called by the close( ) function. But you may want to flush the data before closing any file. The syntax to flush function is :-
<fileobject>.flush( )
Removing Whitespaces after Reading from File
The read( ) and readline( ) functions discussed above, read data from file and return it in a string form and the readlines( ) function returns the entire file content in a list where each line is one item of the list. All these read functions also read the leading and trailing whitespaces i.e., spaces or tab or new line characters.
If you want to remove any of these trailing and leading whitespaces you can use strip( ) function.
- The strip( ) removes the given character from both ends.
- The rstrip( ) removes the given character from trailing end i.e., right end.
- The lstrip( ) removes the given character from leading end i.e., left end.
Important Note:-
Steps to Process a File
- Determine the type of file usage.
- Open the file and assign its reference to a file-object of file-handle.
- Now process as required.
- Close the file.
Working with Binary Files
Objects have some structure and hierarchy associated, it is important that they are stored in way so that their structure/hierarchy is maintained. For this purpose, objects are often serialised and then stored in binary files.
- Serialisation ( also called Pickling ) is the process of converting Python object hierarchy into a byte stream so that it can be written into a file. Pickling converts an object in byte stream in such a way that it can be reconstructed in original form when unpickled or de-serialised.
- Unpickling is the inverse of pickling whereby a byte stream is converted back into an object hierarchy.
“The pickle module implements a fundamental, but powerful algorithm for serialising and de-serialising a Python object structure.”
In order to work with the pickle module you must first import it in your program using import statement.
Import pickle
Process of working with binary files is similar to as you have been doing so far with a little difference that you work with pickle module in binary files i.e.,
- Import pickle module.
- Open binary file in the required file mode (read mode or write mode).
- Process binary file by writing/reading objects using pickle module’s methods.
- Once done, close the file.
NOTE:- You may use dump ( ) and load ( ) methods of pickle module to write and read from an open binary file respectively.
1. Creating/Opening/Closing Binary Files
Binary file is opened in the same way as you open any other file but make sure to be use “b” with file mode to open a file in binary mode e.g.,
Dfile=open("stu.dat","wb+")
Binary file opened in write mode with file-handle as Dfile.
Like text files, a binary file will be get created when opened in an output file mode and it does not exist already. That is, for the file modes, “w”, “w+”, “a”, the file will get created if it does not exist already but if the file exist already, then the file modes “w”, “w+” will overwrite the file and the file mode “a” will retain the contents of file.
An open binary file is closed in the same manner as you close any other file, i.e.,
Dfile.close( )
IMPORTANT:-
If you are opened a binary file in read mode, then the file must exist otherwise an exception ( a runtime error ) is raised. Also in an existing file, when the last record is reached and end of file ( EOF ) is reached, if not handle properly, it may rise EOFError exception. Thus it is important to handle exceptions while opening a file for reading. For this purpose, it is advised to open a file in read mode either in try and except blocks or using with statement.
2. Writing onto Binary File – Pickling
To write an object on the binary file opened in the write mode, you should use dump( ) function of pickle module.
SYNTAX:-
pickle.dump(<object-to-be-written>,<filehandle-of-open-files>)
For Example:-
If you have a file open in handle file1 and you want to write a list namely list1 in the file, then you may write :
pickle.dump(list1,file1)
3. Appending Records in Binary Files
Appending records in binary files is similar to writing, only thing you have to ensure is that you must open the file in append mode(i.e., “ab”). A file opened in append mode will retain the previous records and append the new records in the file.
For Example:-
Write a program to append student records to a file by getting data from user.
import pickle
def append_student_record():
student_record = {}
student_record['name'] = input("Enter student name: ")
student_record['roll_number'] = int(input("Enter roll number: "))
student_record['grades'] = input("Enter grades: ")
with
open('student_records.dat', 'ab') as file:
pickle.dump(student_record, file)
append_student_record()
How the above program is working:-
1. The `append_student_record` function is defined.
2. The function prompts the user to enter the student’s name, roll number, and grades.
3. The entered data is stored in a dictionary called `student_record`.
4. The `with` statement opens the `student_records.dat` file in append binary mode (`’ab’`).
5. The `pickle.dump` function serializes the `student_record` dictionary and writes it to the file.
6. The function is called to append a new student record to the file.
4. Reading from a Binary File – UnPickling
To read from the file using load( ) function of the pickle module as it would then unpickle the data coming from the file.
SYNTAX:-
<object> = pickle.load(<filehandle>)
For Example:-
For instance, to read an object in “nemp” from a file open in file-handle “fout”, you would write:-
nemp = pickle.load(fout)
5. Searching in a File
There are multiple ways to searching for a value stored in a file. The simplest being the sequential search whereby you read the records from a file one by one and then look for the search key in the read record.
In order to search for some value(s) in a file, you need to do the following:-
- Open the file in read mode.
- Read the file contents record by record (i.e., object by object).
- In every read record, look for the desired search-key.
- If found, process as desired.
- If not found, read the next record and look for the desired search-key.
- If search-key is not found in any of the records report that no such value found in the file.
For Example:-
Read file stu.dat and display record having marks > 81.
import pickle
stu={}
found=False
print("Searching in file Stu.dat...")
# open binary file in read mode and process with the with block
with open('Stu.dat', 'rb') as fin:
stu = pickle.load(fin)
if stu['Marks'] > 81:
print(stu)
found = True
if found == False:
print("No records with Marks > 81")
else:
print("Search successful.")
6. Updating in a Binary File
You know that updating an object means changing its value(s) and storing it again. Updating records is a three step process which is –
- Locate the record to be updated by searching for it.
- Make changes in the loaded record in memory (the read record).
- Write back onto the file at the exact location of old record.
Accessing and Manipulating Location of File Pointer —
The two file pointer location functions of Python are : tell( ) and seek( ). These functions work identically with that text files as well as the binary files.
The tell( ) Function
The tell( ) function returns the current position of file-pointer in the file.
SYNTAX:-
<file-handle>.tell()
The seek( ) Function
The seek( ) function changes the position of file-pointer by placing the file-pointer at the specific file position in the open file.
SYNTAX:-
<file-object>.seek(offset[, mode])
Where,
Offset :- is a number specifying number-of-bytes.
Mode :- is a number 0 or 1 or 2 signifying.
- 0 for beginning of file (to move file-pointer w.r.t beginning of file) it is default position (i.e., when no mode is specified)
- 1 for current position of file-pointer (to move file pointer w.r.t current position of it)
- 2 for end of file (to move file pointer w.r.t end of file).
Working with CSV Files
CSV files are delimited files that is store tabular data (data stored in rows and columns as we see in speedsheets or databases) where comma delimits every value, i.e., the values are separated with comma. Each line in CSV file is a data record.
The separator character of CSV files is called a delimiter. Default and most popular delimiter is common. Other popular delimiters include the tab (\t), colon (:), pipe (|) and semi-colon (;).
CSV files are the text files you can apply text file procedures on these and then split value using split( ) function BUT there is a better way of handling CSV files which is– using csv module of Python.
SYNTAX:-
Import csv
1. Opening/Closing CSV Files
A CSV file is opened in the same way as you open any other text file but make sure to do the following two things.
- Specify the file extension as .csv .
- Open the file like other text file.
For Example:-
File1 = open("stu.csv","r")
In above example CSV file opened in read mode with file-handle as File1.
Like text files, a CSV file will get created when opened in an output file mode and if it does not exist already. That is, for the file modes, “w”, “w+”, “a”, “a+” , the file will get created if it does not exist already and if it exist already, then the file mode “w” and “w+” will overwrite the existing file and the file mode “a” or “a+” will retain the contents of the file.
For Closing the CSV files you have to write–
<file-handle>.close()
NOTE:-
The CSV files are popular because of these reasons:-
- Easier to create.
- Preferred export and import format for databases and spreadsheets.
- Capable of storing large amounts of data.
2. Writing in CSV Files
Writing into CSV Files involves the conversion of the user data into the writable delimited form and then stored it in the form of CSV file. For writing onto a CSV files you normally use the following three functions.
- csv.writer( ) :– returns a writer object which writes data into CSV file.
- <writerobject>.writerow( ) :– writes one row of data onto the writer object.
- <writerobject>.writerows( ) :– writes multiple rows of data onto the writer object.
3. Reading in CSV Files
By using csv.reader( ) function. The csv.reader object does the opposite of csv.writer object. The csv.reader object loads data from the csv file, parses it, i.e., removes the delimiters and returns the data in the form of a Python iterable wherefrom you can fetch one row of data at a time.
To read from a CSV file, you need to do the following:–
- import csv module.
- Open csv file in a file-handle in read mode (just as you open other text files).
- Create the reader object by using the syntax.
- The reader object store the past data in the form of iterable and thus you can fetch from it row by row through a traditional for loop, one row at a time.
- Process the fetched single row of data as required.
- One done, close the file.
For Example:-
Write a program to read and display the contents of Employee.csv file.
import csv
with open("Employee.csv", "r") as fh:
ereader = csv.reader(fh)
print("File Employee.csv contains:")
for rec in ereader :
print(rec)
Last Word :-May the radiance of this blog post have enchanted your souls. Gratitude for your unwavering support and affection. Await our next encounter with a fresh blog, as you continue to journey through realms of discovery, we will help you.